The Local Interconnect Network (LIN) protocol specification provides a
low-cost, short-distance, and low-speed network, enabling the
implementation of a new level of electronics intelligence in automotive
subsystems.
LIN operates under a CAN platform, but it doesn't require the robust
data rate and bandwidth performance, or the higher cost, associated with
CAN.
Depending on the speed and cost requirements, either CAN or LIN can be implemented.
LIN is a single-master multislave bus that communicates via a single
wire, reducing wiring complexity as well as cost. Because this protocol
is self-synchronizing, it allows the slave nodes to run from a low-cost
RC oscillator.
Embedded and Network Software Programmer Concepts
Tuesday, 29 January 2019
C++ Deleted and default
default
If a class is defined with any constructors, the compiler will not generate a default constructor. This is useful in many cases, but it is some times vexing. For example, we defined the class as below:
class A { public: A(int a){}; };
Then, if we do:
A a;
The compiler complains that we have no default constructor. That's because compiler did not make the one for us because we've already had one that we defined.
We can force the compiler make the one for us by using default specifier:class A
{
public:
A(int a){}
A() = default;
};
Then, compiler won't complain for this any more:
A a;
delete
Suppose we have a class with a constructor taking an integer:
class A { public: A(int a){}; };
Then, the following three operations will be successful:
A a(10); // OK A b(3.14); // OK 3.14 will be converted to 3 a = b; // OK We have a compiler generated assignment operator
However, what if that was not we wanted. We do not want the constructor allow double type parameter nor the assignment to work.
C++11 allows us to disable certain features by using delete:
class A { public: A(int a){}; A(double) = delete; // conversion disabled A& operator=(const A&) = delete; // assignment operator disabled };
Then, if we write the code as below:
A a(10); // OK A b(3.14); // Error: conversion from double to int disabled a = b; // Error: assignment operator disabled
In that way, we could achieve what we intended.
Thursday, 30 August 2018
CPLD Vs FPGA
A complex programmable logic device (CPLD) is a logic device with completely programmable AND/OR arrays and macrocells.
A complex programmable logic device is an innovative product compared to earlier logic devices like programmable logic arrays (PLAs) and Programmable Array Logic (PAL). The earlier logic devices were not programmable, so the logic was built by combining multiple logic chips together. A CPLD has a complexity between PALs and field-programmable gate arrays (FPGAs).
A complex programmable logic device is an innovative product compared to earlier logic devices like programmable logic arrays (PLAs) and Programmable Array Logic (PAL). The earlier logic devices were not programmable, so the logic was built by combining multiple logic chips together. A CPLD has a complexity between PALs and field-programmable gate arrays (FPGAs).
CPLD vs FPGA comparison summary
No. | CPLD | FPGA |
---|---|---|
1 | Instant-on. CPLDs start working as soon as they are powered up | Since FPGA has to load configuration data from external ROM and setup the fabric before it can start functioning, there is a time delay between power ON and FPGA starts working. The time delay can be as large as several tens of milliseconds. |
2 | Non-volatile. CPLDs remain programmed, and retain their circuit after powering down. FPGAs go blank as soon as powered-off. | FPGAs uses SRAM based configuration storage. The contents of the memory is lost as soon as power is disconnected. |
3 | Deterministic Timing Analysis. Since CPLDs are comparatively simpler to FPGAs, and the number of interconnects are less, the timing analysis can be done much more easily. | Size and complexity of FPGA logic can be humongous compared to CPLDs. This opens up the possibility less deterministic signal routing and thus causing complicated timing scenarios. Thankfully implementation tools provided by FPGA vendors have mechanisms to assist achieving deterministic timing. But additional steps by the user is usually necessary to achieve this. |
4 | Lower idle power consumption. Newer CPLDs such as CoolRunner-II use around 50 uA in idle conditions. | Relatively higher idle power consumption. |
5 | Might be cheaper for implementing simpler circuits | FPGAs are much more capable compared to CPLDs but can be more expensive as well. |
6 | More "secure" due to design storage within built in non-volatile memory. | FPGAs that use external memory can expose the IP externally. Many FPGA vendors offer mechanisms such as encryption to combat this. Design specific protection mechanisms also can be implemented. |
7 | Very small amount of logic resources. | Massive amount logic and storage elements, with which incredibly complex circuits can be designed. FPGAs have thousands times more resources! This point alone makes FPGAs more popular than CPLDs. |
8 | No on-die hard IPs available to offload processing from the logic fabric. | Variety of on-die dedicated hardware such as Block RAM, DSP blocks, PLL, DCMs, Memory Controllers, Multi-Gigabit Transceivers etc give immense flexibility. This is not even thinkable with CPLDs. |
9 | Power down and reprogramming is always required in order to modify design functionality. | FPGAs can change their circuit even while running! (Since it is just a matter of updating LUTs with different content) This is called Partial Reconfiguration, and is very useful when FPGAs need to keep running a design and at the same time update the it with different design as per requirement. This feature is widely used in Accelerated Computing. |
Where to use CPLD or FPGA
When a design requires simple glue-logic or similar functionality which doesn’t need to be changed much, or when you need an instant-on circuit, then go for CPLDs. Otherwise, for most other applications FPGAs are generally preferred. Sometimes you can find both CPLD + FPGA in a design. In those designs, CPLDs generally do simple glue-logic as mentioned before, and are responsible for “booting” the FPGA as well as controlling reset and boot sequence of complete board.
Monday, 6 August 2018
Depedency UML
a dependency is displayed in the diagram editor as a dashed line with an open arrow that points from the client to the supplier.

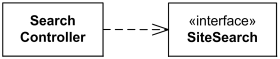
Class SearchController depends on (requires) SiteSearch interface.
In this case, the dependency is an instantiate dependency, where the Car class is an instance of the CarFactory class."

Wrong: Car class has a dependency on the CarFactory class.
Right: CarFactory class depends on the Car class.
Right: CarFactory class depends on the Car class.
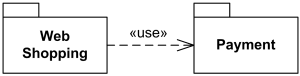
Web Shopping package uses (depends on) Payment package.
Create is a usage dependency denoting that the client classifier creates instances of the supplier classifier. It is denoted with the standard stereotype «create».

Class DataSource creates Connection.

Generalization

Association
that conceptually means that the two components are linked to each other.
This association is represented by the following symbol in UML:


Friday, 3 August 2018
extend and include in UML
Include = reuse of functionality
Extends = new and/or optional functionality
Extends = new and/or optional functionality
The include relationship allows one use case to include the steps of another use case.
For example, suppose you have an Amazon Account and you want to check on an order, well it is impossible to check on the order without first logging into your account. So the flow of events would like so...
The extend relationship is used to add an extra step to the flow of a use case, that is usually an optional step...
Imagine that we are still talking about your amazon account. Lets assume the base case is Orderand the extension use case is Amazon Prime. The user can choose to just order the item regularly, or, the user has the option to select Amazon Prime which ensure his order will arrive faster at higher cost.
Sunday, 29 July 2018
Rhapsody Basics
A Rhapsody Unit is a file system representation of modeling elements such as projects, packages, use case diagrams, object model diagrams, components, etc.
Each unit has a specific extension depending on its type:
Table 1. Unit types and corresponding file extension
Type | File Extension |
---|---|
Project | .rpy |
Packages / profiles | .sbs |
Object model diagrams | .omd |
Structure diagrams | .std |
Sequence diagrams | .msc |
Components | .cmp |
Use case diagrams | .ucd |
Classes, actors & use cases | .cls |
Collaboration diagrams | .clb |
Component diagrams | .ctd |
Deployment diagrams | .dpd |
Panel diagrams | .pld |
Controlled files | any extension |
Sunday, 22 July 2018
Linux Daemon Process
#include#include #include #include #include #include #include #include #include int main(void) { /* Our process ID and Session ID */ pid_t pid, sid; /* Fork off the parent process */ pid = fork(); if (pid < 0) { exit(EXIT_FAILURE); } /* If we got a good PID, then we can exit the parent process. */ if (pid > 0) { exit(EXIT_SUCCESS); } /* Change the file mode mask */ umask(0); /* Open any logs here */ /* Create a new SID for the child process */ sid = setsid(); if (sid < 0) { /* Log the failure */ exit(EXIT_FAILURE); } /* Change the current working directory */ if ((chdir("/")) < 0) { /* Log the failure */ exit(EXIT_FAILURE); } /* Close out the standard file descriptors */ close(STDIN_FILENO); close(STDOUT_FILENO); close(STDERR_FILENO); /* Daemon-specific initialization goes here */ /* The Big Loop */ while (1) { /* Do some task here ... */ sleep(30); /* wait 30 seconds */ } exit(EXIT_SUCCESS); }
---------------
1) The fork() function returns either the process id (PID) of the child process (not equal to zero), or -1 on failure. If the process cannot fork a child, then the daemon should terminate right here.
2) In order to write to any files (including logs) created by the daemon, the file mode mask (umask) must be changed to ensure that they can be written to or read from properly. By setting the umask to 0, we will have full access to the files generated by the daemon. Even if you aren't planning on using any files, it is a good idea to set the umask here anyway, just in case you will be accessing files on the filesystem.
3)From here, the child process must get a unique SID from the kernel in order to operate. Otherwise, the child process becomes an orphan in the system. Again, the setsid() function has the same return type as fork().
4)One of the last steps in setting up a daemon is closing out the standard file descriptors (STDIN, STDOUT, STDERR). Since a daemon cannot use the terminal, these file descriptors are redundant and a potential security hazard.
Saturday, 21 July 2018
Kernel Thread
Process
An executing instance of a program is called a process. Some operating systems use the term ‘task‘ to refer to a program that is being executed. Process is a heavy weight process. Context switch between the process is time consuming.
Threads
A thread is an independent flow of control that operates within the same address space as other independent flows of control within a process.
One process can have multiple threads, with each thread executing different code concurrently.
Some of the advantages of the thread, is that since all the threads within the processes share the same address space, the communication between the threads is far easier and less time consuming as compared to processes. This approach has one disadvantage also. It leads to several concurrency issues and require the synchronization mechanisms to handle the same.
Types of Thread
There are two types of thread.
- User Level Thread
- Kernel Level Thread
User Level Thread
In this type, kernel is not aware of these threads. Everything is maintained by the user thread library. That thread library contains code for creating and destroying threads, for passing message and data between threads, for scheduling thread execution and for saving and restoring thread contexts. So all will be in User Space.
Kernel Level Thread
Kernel level threads are managed by the OS, therefore, thread operations are implemented in the kernel code. There is no thread management code in the application area.
kthread_create
create a kthread.
struct task_struct * kthread_create (int (* threadfn(void *data), void *data, const char namefmt[], ...);
Where,
threadfn – the function to run until signal_pending(current).data – data ptr for threadfn.namefmt[] – printf-style name for the thread.... – variable arguments |
Start Kernel Thread
wake_up_process
This is used to Wake up a specific process.int wake_up_process (struct task_struct * p);
Where,
p – The process to be woken up.
Attempt to wake up the nominated process and move it to the set of runnable processes.
It returns 1 if the process was woken up, 0 if it was already running.
|
Stop Kernel Thread
kthread_stop
It stops a thread created by kthread_create .int kthread_stop ( struct task_struct *k);
Where,
k – thread created by kthread_create . |
Other functions in Kernel Thread
kthread_should_stop
should this kthread return now?
int kthread_should_stop (void);
When someone calls
kthread_stop on your kthread, it will be woken and this will return true. You should then return, and your return value will be passed through to kthread_stop . |
kthread_run
This is used to create and wake a thread.
kthread_run (threadfn, data, namefmt, ...);
Where,
threadfn – the function to run until signal_pending(current).data – data ptr for threadfn.namefmt – printf-style name for the thread.... – variable arguments |
Example:
static struct task_struct *etx_thread
int thread_function(void *pv)
{
int i=0;
while(!kthread_should_stop()) {
printk(KERN_INFO "In EmbeTronicX Thread Function %d\n", i++);
msleep(1000);
}
return 0;
}
etx_thread = kthread_create(thread_function,NULL,"eTx Thread");
if(etx_thread) {
wake_up_process(etx_thread);
} else {
printk(KERN_ERR "Cannot create kthread\n");
goto r_device;
}
Subscribe to:
Posts (Atom)