When is user defined copy constructor needed?If we don’t define our own copy constructor, the C++ compiler creates a default copy constructor for each class which does a member wise copy between objects. The compiler created copy constructor works fine in general. We need to define our own copy constructor only if an object has pointers or any run time allocation of resource like file handle, a network connection..etc.
Deep copy is possible only with user defined copy constructor. In user defined copy constructor, we make sure that pointers (or references) of copied object point to new memory locations.
Deep copy is possible only with user defined copy constructor. In user defined copy constructor, we make sure that pointers (or references) of copied object point to new memory locations.
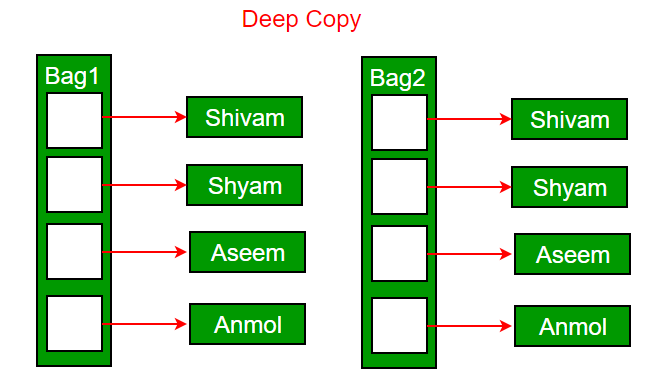
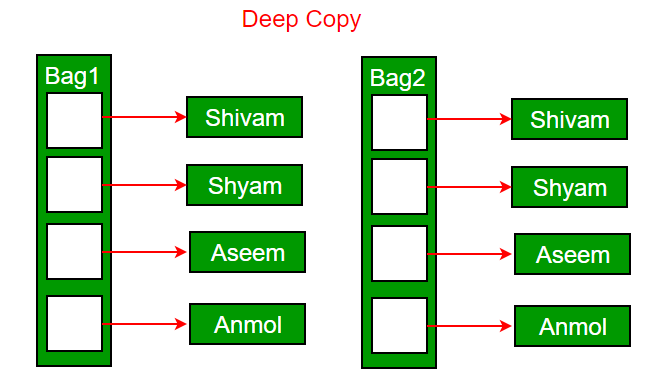
class
String
{
private
:
char
*s;
int
size;
public
:
String(
const
char
*str = NULL);
// default constructor
~String() {
delete
[] s; }
// destructor
String(
const
String&);
// copy constructor
void
print() { cout << s << endl; }
// Function to print string
void
change(
const
char
*);
// Function to change
};
//Copy constructor
String::String(
const
String& old_str)
{
size = old_str.size;
s =
new
char
[size+1];
strcpy
(s, old_str.s);
}
Point(int x1, int y1) { x = x1; y = y1; }
// Copy constructor
Point(const Point &p2) {x = p2.x; y = p2.y; }
When is copy constructor called?
In C++, a Copy Constructor may be called in following cases:
- When an object of the class is returned by value.
- When an object of the class is passed (to a function) by value as an argument.
- When an object is constructed based on another object of the same class.
- When compiler generates a temporary object.
Can we make copy constructor private?
Yes, a copy constructor can be made private
No comments:
Post a Comment